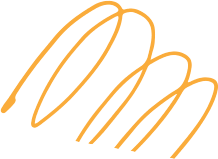
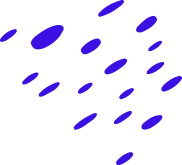
Short Overview
C is a general-purpose programming language created by Dennis Ritchie at the Bell Laboratories in 1972. It is a very popular language, despite being old. C is strongly associated with UNIX, as it was developed to write the UNIX operating system.
- Course Overview
- Introduction of C language
- Why should we learn the C language ?
- When it launched ?
- Who launched it ?
- Where it was launched ?
- Why it named C ?
- Why it is so popular ?
- Writing the source code
- Compilation the source code
- Execution
- Compiler
- Interpreter
- Assembler
- What is an IDE ?
- Full Form of IDE
- Which IDE we will use ?
- Writing the source code
- Compilation of the code
- Execution / running
- Introduction to Turbo C++
- IIntroduction to Header files
- What are Header files
- Introduction to stdio.h
- Introduction to pond
- Introduction to preprocessor directive
- What is console window
- Introduction to conio.h
- How the header files work
- int main
- void main
- main
- Importance of main
- What is program
- Compilation of the code
- What is function
- Syntax of program
- print f()
- scan f()
- How the code compile
- Types of compiler
- What happen when we compile a program
- What is syntax error
- How the program run
- What happen when the program runs
- What is getch()
- Using getch()
- How getch() works
- What are escape sequences
- Using escape sequences
- Displaying special character
- Variables
- Data types
- Changing the default precision
- Rules of declaring variables
- Types of data types
- ASCII
- Types of operator
- Precedence and associativity of operators
- Developing programs in C
- Type casting
- Type conversion
- Difference between type casting and type conversion
- Type promotion
- Increment decrement operators
- if
- else
- if else if else
- switch
- Ternary operator
- goto
- Introduction to Loop
- Types of loop
- While
- Do While
- For
- Various forms of loop
- Nested loop
- Break
- Size of
- continue
- typedef
- Single dimensional integer array
- How do we access array elements
- Printing array data
- Accepting input from the user in the array
- Initialization of an array
- Sorting the array
- Double dimensional integer array
- Single dimensional character array(string)
- Initialization of an character array
- Traversing the character array
- Functions in header file string.h
- What is function
- Advantages of using functions
- Types of Functions
- How to configure our system for database programming.
- Steps required for developing a function
- Some important facts about main
- Prototype of function
- Components of function declaration
- Library files
- Understanding the prototype of function malloc()
- What is a pointer
- How to create pointer
- Dereferencing of pointers
- Indirect addressing
- Accessing array using pointer
- Traversing a character array
- Important rule regarding pointers
- Pass by reference
- Pass by value
- Relation between array and pointer
- What is dynamic memory allocation
- Types of memory allocation
- How DMA done in C language
- Void pointer
- About structure
- Terminologies used in structure
- Using initializers with structure creating array of structure
- Structure and pointer
- Structure and functions
C Programming Lecture - 1.1 | Preview |
C Programming Lecture - 1.2 | Preview |
C Programming Lecture - 1.3 | Preview |
C Programming Lecture - 2.1 | Preview |
C Programming Lecture - 2.2 | Preview |
C Programming Lecture - 2.3 | Preview |
C Programming Lecture - 3.1 | Preview |
C Programming Lecture - 3.2 | Preview |
C Programming Lecture - 3.3 | Preview |
From Fundamentals to Advanced C Programming
- C Basics to Advanced: Learn C programming from fundamental concepts to advanced techniques.
- Zero to Hero: Evolve from a beginner to a proficient C programmer.
- Core Concepts to Advanced Topics: Cover everything from C basics to advanced topics like pointers and memory management.
Live Lectures
- 60 Hours: Engage in live, interactive sessions led by experienced C developers.
- Hands-on Practice: Enhance your C programming skills through coding exercises during live lectures.
- Practice Sessions: Participate in dedicated sessions to fully grasp and apply C programming concepts.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Clear your doubts with help from seasoned instructors.
Test Series and Assignments
- Topic-Wise Test Series: Test your understanding with assessments after each significant topic.
- Assignments: Apply your learning through practical C programming assignments.
Lecture Notes
- Comprehensive Notes: Access detailed notes for every lecture, covering critical C programming topics.
Projects
- Real-World Projects: Implement your C programming skills on live projects, simulating real-world scenarios.
- Data Structures and Algorithms: Build and optimize projects using advanced data structures and algorithms.
- Best Practices: Learn to follow industry-standard best practices in your C programming projects.
Certifications
- Dual Certification: Obtain two certificates upon course completion and project submission.
- C Programming Completion Certificate: Receive a certificate for successfully completing the C programming course.
- Project Completion Certificate: Get certified for successfully delivering your C programming project.
Computer / Laptop Requirments
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
Text Editor/IDE
- Visual Studio Code: A lightweight code editor with extensions for C programming.
- Code::Blocks: An open-source IDE with built-in C support.
- Dev-C++: A lightweight IDE for C and C++ programming.
- Eclipse IDE for C Developers: A versatile IDE with C development capabilities.
Compiler
- GCC (GNU Compiler Collection): A standard and widely used open-source compiler for C.
- MinGW (Minimalist GNU for Windows): A Windows port of the GCC compiler.
- Clang: A compiler for C language that works on macOS and Linux, providing fast compilation times.
Debugger
- GDB (GNU Debugger): A widely used debugger that comes bundled with GCC.
- Integrated Debuggers in IDEs: Visual Studio Code, Code::Blocks, and Eclipse IDE have built-in debugging tools.
Build Tools (Optional)
- Make: A build automation tool, often used with GCC.
- CMake: A cross-platform build system generator commonly used in C development.
Version Control
Ready to kick-start your career?
Join Us Now Join Us Now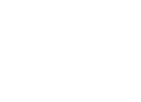
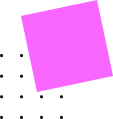