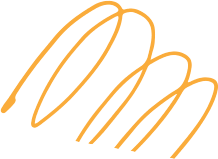
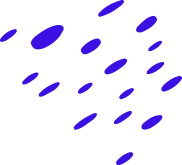
Short Overview
React is a free and open-source front-end JavaScript library for building user interfaces based on components. It is maintained by Meta and a community of individual developers and companies
- Introduction to HTML
- History of HTML
- Components of HTML
- Structure of HTML
- Body section
- Categories of Tag in Body Section
- How to display images
- Creating List
- Ordered List
- Unordered List
- Definition List
- How to work with background?
- How to print special characters?
- Creating Tables in HTML
- Attributes of Table
- Generating Irregular Tables
- How to work with forms?
- How to work input controls?
- Using <select> Tag
- Generating Drop Down List
- Generating Scrollable List
- How to work text area?
- Introduction to HTML 5
- Improvements Given by HTML 5
- Web Forms 2.0
- New Input Elements
- New Form Attributes
- DataList Tag
- Playing Audio
- Controlling Audio
- Playing Video
- Introduction to CSS
- Advantages of CSS
- Versions of CSS
- Versions of CSS
- Types of CSS
- CSS Selectors
- Project
- Inheritance in CSS
- CSS Units
- Absolute v/s Relative Units
- Styling Background using CSS
- Handling Font in CSS
- Various Font Properties
- What are Google Fonts
- How to use Google Fonts
- Project
- Handling Text in CSS
- Various Text Properties
- Removing Underline from Links
- Handling Link in CSS
- Pseudo-Classes
- Making Links Look Like Button
- Styling Lists in CSS
- Making Nav Bar Using List
- Styling Tables in CSS
- Styling Table Border
- Creating Zebra Striped Tables
- Making Tables Responsive
- Project
- The <div> and <span> Tags
- The Difference
- Introduction to semantic HTML
- Popular Semantic HTML Tags
- The CSS Box Model
- Border, Padding and Margin
- Handling Overflow
- Setting Element Pos using Float
- The Visibility Property
- The Display Property
- Positioning in CSS
- Various Types of Positioning in CSS
- Other properties used with Positioning
- Styling form in CSS
- Attribute Selectors
- Styling Controls
- What is JavaScript?
- Who runs our JavaScript code?
- Relationship with HTML and CSS
- What JavaScript can do in Browser?
- What JavaScript can't do?
- Java v/s JavaScript
- History of JavaScript
- JavaScript Embedding
- Writing to the document
- Using Browser Console
- Comments in JavaScript
- Declaring variables in JavaScript
- Data Types
- Primitive Data Types and Non-Primitive Data Types
- Difference between null and undefined
- Var v/s let
- Types of Dialog Box
- Accepting Numeric input through Dialog box
- Using Template String
- Types of Operators
- Type Conversion & Type Coercion
- Standard rules of Number Conversion
- Controlling Decimal Points
- If
- If else
- If else if else
- Nested if
- Ternary Operator
- Switch
- While loop
- Do while loop
- For loop
- For/of loop
- For/in loop
- Array Definition
- Creating Array
- Using Loop with array
- Array with mixed type
- Creating Array using Constructor
- Array Methods
- What is Function?
- Function calling
- Calling Function from link
- Variable scope
- Returning values
- Calling function via Button
- Anonymous Functions
- Some important built-in functions
- Objects in JavaScript
- Creating Objects
- Adding properties, changing properties, and Deleting properties
- Adding methods
- Nested Objects
- Creating object using object literal
- The toString() method
- Overriding toString()
- Object equality in JavaScript
- What is JSON?
- The 'this' keyword and using of 'this' keyword
- The Arrow function
- Restriction on Arrow function
- Callback function
- Anonymous function as callbacks
- Why we need callback?
- The Date object
- The String object
- The Math object
- DOM introduction
- What is DOM?
- What can be done with DOM?
- DOM selection methods
- Setting/Getting Text Content
- Changing CSS
- Adding & Removing classes
- Traversing the DOM
- DOM properties
- DOM properties drawback
- Modern DOM properties
- Creating new element
- append() & preprend()
- Event Introduction
- Event categories
- Ways of handling events
- Using function for event handling
- Cancelling the default behaviour
- Using DOM event handler
- Working with MouseEvents
- Working with KeyBoard Events
- Determining the key Pressed
- Using DOM level 2 event handler
- The event object
- Event Bubbling
- Event delegation
- Accessing form object through JavaScript
- Accessing Form data
- The submit event & how to handle submit event
- Other form events
- Creating A ToDo List App
- Apply All The Concepts Learnt Till Now
- What Is React ?
- What Problem React Solves ?
- What Are Single Page Application’s ?
- Challenges In Developing A SPA
- React Advantages.
- Is React A Framework OR Library ?
- Two Ways To Develop A React App.
- Developing Using CDN.
- Developing Using Create React App.
- Difference between CDN And Create React App
- Developing The First App
- Creating Function Based React Element
- Creating Siblings
- Creating Nested Components
- What is JSX ?
- Why JSX ?
- Characteristics Of JSX ?
- Coding JSX
- Expressions In JSX
- Inserting A Large Block Of HTML
- Conditional Rendering
- Different Ways Of Conditional Rendering
- Modules
- Exports and Imports
- Classes
- Spread & Rest Operators
- Destructuring
- Two Types Of Components
- Class Components
- How To Create Class Components
- Industry Recommended Way Of Structuring A React Application
- What are props ?
- Using Props In React
- Some Important Points About Props
- How To Iterate Over Arrays In JSX
- Using map() Method Of Array
- Default props
- Styling In React
- Different Ways Of Styling
- Inline Styling
- JS Object Styling
- Introduction To CRA
- Installation Of Node Package Manager
- Creating A New Project Using Create-React-App
- Introduction To CRA
- Installation Of Node Package Manager
- Creating A New Project Using Create-React-App.
- Exercise To Better Understand Request Flow
- CRA Conventions
- How To Load Images In CRA ?
- How To Add CSS Files In CRA ?
- What Is State ?
- How It Is Different Than Props ?
- Different Ways Of Initializing State
- Why To Update The State ?
- Correct Way To Update The State
- Points To Remember
- Event Handling Basics
- How React's Event Handling Differs From JS ?
- Concept Of Binding
- Passing Arguments To Event Handlers
- Different Ways Of Passing Arguments
- React Keyboard Events
- Getting The Key Pressed
- React Design Principles
- Recommended Way Of State Updation
- What To Keep In State ?
- Data Flow In State
- React Design Principles
- Recommended Way Of State Updation
- What To Keep In State ?
- Data Flow In State
- Form Recap
- How Forms Work In React ?
- Uncontrolled Components V/s Controlled Components
- Adding Multiple Fields
- Creating Dynamic Keys
- Modifying The ToDo List App Using React
- Apply All The Concepts Learnt Till Now
- Uncontrolled Components
- Creating Ref
- Accessing Ref
- TextArea In HTML
- TextArea In React
- DropDown List In HTML
- DropDownList In React
- React Component Life Cycle Phases
- React Component Life Cycle Methods
- Mounting Phase Methods
- Updating Phase Methods
- Unmounting Phase Methods
- What Is A Fragment ?
- Need Of A Fragment
- Syntax
- Introduction
- What Is localStorage ?
- Methods Of localStorage
- Introduction To Hooks
- What Exactly Are Hooks ?
- Rules Of Hooks
- Types Of Hooks
- Introduction To useState() Hook
- Syntax Of useState()
- Introduction To useEffect()
- Syntax
- Use Of Dependencies Argument
- Performing Clean Up
- Introduction To Context API In React
- The useContext() Hook
- Project
- Introduction To Reducer In JS
- The reduce() Method
- Understanding useReducer() Hook
- Project
- Multi page React sites
- React Router Setup
- Links and Nav Links
- Switch and exact match
- Fetching Data
- Route Parameters
- Introduction to React Router 6
- The Route Component
- Redirects and use Navigate
- Fetch API in React to populate data
From Fundamentals to Advanced React JS
- React Basics to Advanced: Master React JS from the ground up, covering fundamental to advanced concepts.
- Zero to Hero: Transition from a novice to a skilled React developer.
- Core Concepts to Advanced Patterns: Explore everything from React basics to advanced patterns and state management.
Online and Offline Lectures
- 90 Hours: Participate in online and offline, interactive sessions led by industry professionals.
- Hands-on Practice: Strengthen your React skills through coding exercises during live sessions.
- Practice Sessions: Join dedicated sessions to thoroughly understand and apply React concepts.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Get all your questions answered by experienced instructors.
Test Series and Assignments
- Topic-Wise Test Series: Assess your understanding with tests after each key topic.
- Assignments: Solidify your knowledge by completing practical coding assignments.
Lecture Notes
- Detailed Notes: Access comprehensive notes for every lecture, covering essential React JS topics.
Projects
- Real-World Projects: Work on live projects to apply React concepts in practical scenarios.
- Component-Based Architecture: Learn to design and build scalable React applications using best practices.
- Advanced State Management: Master state management in React using Context API, Redux, and other tools.
Certifications
- Dual Certification: Receive two certificates upon course completion and project delivery.
- React Completion Certificate: Earn a certificate for completing the React JS course.
- Project Completion Certificate: Get certified for successfully completing your React project.
Computer / Laptop Requirments
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
Text Editor/IDE
- Visual Studio Code: A widely used code editor, ideal for React JS development.
- WebStorm: A robust IDE designed for JavaScript development.
- Sublime Text: A lightweight and customizable text editor.
Node.js and npm
- Node.js: The JavaScript runtime that allows you to run JS outside the browser, essential for React's development.
- npm (Node Package Manager): Installed along with Node.js. It’s used for managing dependencies and packages in React projects.
- Yarn (optional): An alternative package manager to npm.
React.js
- React.js: The JavaScript library for building user interfaces. Installed via npm with
npx create-react-app
ornpm install react
.
Installing React using Vite
Vite is a modern development build tool. To create a React app with Vite:
npm create vite@latest my-react-app --template react
cd my-react-app
npm install
npm run dev
- Vite: A fast and optimized build tool for React applications.
Installing React using Parcel
Parcel is another modern, zero-config build tool. To set up React with Parcel:
mkdir my-react-app
cd my-react-app
npm init -y
npm install react react-dom
npm install --save-dev parcel
Create index.html
and index.js
, then run:
npx parcel index.html
- Parcel: A powerful, zero-config bundler for web applications.
Version Control System
- Git: For tracking changes in your React projects. Use with GitHub, GitLab, or Bitbucket.
- Git Extensions (optional): A GUI tool for Git.
Browser
- Google Chrome: A modern browser with excellent developer tools.
- Mozilla Firefox: Another great browser with useful development tools.
Browser Developer Tools
- React Developer Tools: A Chrome/Firefox extension that allows you to inspect the React component hierarchy in the browser’s Developer Tools.
Code Linters and Formatters (Optional)
- ESLint: A tool for identifying and fixing problems in JavaScript code.
- Prettier: An opinionated code formatter to maintain consistent code style.
State Management (Optional)
- Redux: A popular state management library for React applications.
- React Context API: Built-in functionality in React for state management in smaller applications.
Testing Tools
- Jest: A popular testing framework for JavaScript, often used with React.
- React Testing Library: A lightweight library for testing React components.
API Testing Tools (Optional)
- Postman: A powerful tool for testing APIs.
- Insomnia: Another API testing tool for interacting with backends.
Build Tools
- Webpack: A module bundler for bundling JavaScript files for use in a browser.
- Babel: A JavaScript compiler used to convert modern JS code into versions compatible with older browsers.
Deployment Tools
Ready to kick-start your career?
Join Us Now Join Us Now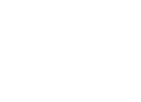
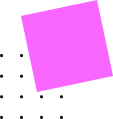