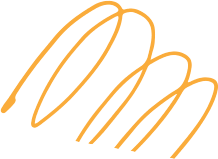
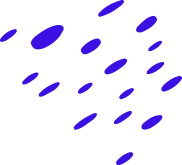
Short Overview
The Java SE is a computing-based platform and used for developing desktop or Window based applications. Thus, core Java is the part of Java SE where the developers develop desktop-based applications by using the basic concepts of Java where JDK (Java Development Kit) is a quite familiar Java SE implementation.
- Prerequisites of Leaning of Java
- Necessity of Learning Java
- Brief History of Java
- What is Java ?
- What is Platform Independent ?
- Where Java stands today ?
- Platform Independent
- Automatic memory management
- Secure
- Robust
- Simplicity
- Multithreaded
- History of Java
- Edition of Java
- Difference between JDK , JRE and JVM
- Downloading JDK
- Installing JDK
- Verifying The installing
- Different ways of writing a Java code
- Introduction of main()
- Why main() is public ?
- Why main() is static ?
- What is String [ ] args ?
- Understanding System.out.println()
- Saving the source code
- Compilation process and it's explanation
- Executing the code
- Discussion About some important Errors
- Brief introductions of import & java.lang
- Data types
- Primitive & Non primitive data types
- Type Conversion
- Implicit Type Conversion
- Explicit Type Conversion
- Type Conversion in expression
- Introduction of operators
- Displaying values of variables
- Concatenating using '+' operator
- Concept of Default values
- Using 'Math' class
- Program to calculate circumference and Area of circle
- Various ways of accepting input
- Accepting input through Command Line Argument
- Wrapper Classes
- Uses of wrapper classes
- Converting string to primitive
- Why did Exception occur when accepting input in some cases ?
- Scanner Class
- Accepting String & Integers
- InputMismatchException
- Concepts of object & object reference
- Introduction of String in java
- Creating object of String
- Important methods of String
- Concatenating String
- Discussion of decision control statement
- If, If - else, nested if
- Switch
- Ternary operator
- Loop structure and it's types
- while and do while loop
- for loop
- Labeled break and continue
- Nested loop
- Arrays (Types & Syntax)
- How JVM handles dynamic blocks ?
- Garbage Collector
- Enhanced for loop
- Drawback of enhanced loop
- Two dimensional arrays
- Rectangular 2D array
- Jagged 2D Array
- Objects And classes
- Pillars of OOP
- Creating a class and its object
- Access modifiers
- Creating methods in class
- Initialising data members of the class
- Creating parametrised method in a class
- Initialising Object or Data members
- Explicit initialisation
- Using constructor
- Parametrised constructor
- Arguments passing
- Passing variables
- Passing array reference
- Returning array reference
- Using “this” keyword
- Using the keyword “static”
- static data members
- Garbage collector
- The 'Object' class
- Using “static” methods
- Properties of “static” methods
- Factory methods
- “static” block
- Types of Inheritance
- Using keyword “super”
- Constructor calling in inheritance
- Calling parametrised constructor in inheritance
- Calling non-parametrised constructor in inheritance
- Method Overriding
- Overloading vs Overriding
- Relationship between base class reference & derived class object
- Polymorphism dynamic method dispatch
- Binding
- Abstract classes and methods
- Using “final” keyword
- final data members
- final methods
- final classes
- Interfaces
- What's new in interface
- Interface with runtime polymorphism
- Inheriting one interface into another
- Packages
- Benefits of packages
- Creating packages inside the bin or outside the bin
- Setting path & classPath
- Access modifiers or visibility modes
- Using static import
- Solving ambiguity in packages
- What is Exception handling
- Exception handling keywords
- try and catch
- Exception hierarchy
- Obtaining description of an Exception
- Using the keyword “throw”
- Checked and Unchecked Exception
- Creating programmer defined exception / custom exception
- Using the keyword “finally”
- Multiple catch feature in java
- String handling
- Constructors & methods of String class
- StringBuffer
- Constructors & methods of StringBuffer class
- File Handling
- Operations on File
- The File class
- Constructors & methods of File class
- The FileReader class
- The BufferedReader class
- The Writer class
- What is Collection ?
- Advantages of Collections
- Array vs Collections
- Types of Collections
- Hierarchy of Collection
- Important methods of Collections
- Collection vs Collections
- The “ArrayList” Class
- Type un safe And Type safe ArrayList
- Operation of ArrayList
- Introduction & Creation of Custom ArrayList
- The “LinkedList” Class
- Internal Mechanisms of LinkedList
- Operation of LinkedList
- The “HashSet” Class
- Operation of HashSet
- What is an Iterator ?
- Methods of Iterator
- Working of an Iterator
- Why duplicates were not removed ? ( Concept of Hashcode )
- The “TreeSet” Class
- Introduction & Adding custom object to TreeSet
- Methods of TreeSet
- HashSet vs TreeSet
- Important methods of Map
- Important methods of Entry
- The “HashMap” Class
- Constructor of HashMap
- Adding & retrieving data in HashMap
- Methods of HashMap
- The “TreeMap” Class
- Adding & retrieving data in TreeMap
- Methods of TreeMap
- HashMap vs TreeMap
From Fundamentals to Advanced Core Java (JSE)
- Java Basics to Advanced: Master Core Java (Java Standard Edition) from foundational concepts to advanced topics.
- Zero to Hero: Develop your skills from a beginner to a proficient Core Java developer.
- Comprehensive Coverage: Learn everything from object-oriented programming (OOP) to multithreading, collections, and exception handling in Java.
Live Lectures
- 60 Hours: Engage in live, interactive sessions led by experienced Java instructors.
- Hands-on Practice: Strengthen your Java skills through practical coding exercises during live sessions.
- Practice Sessions: Join dedicated sessions to fully understand and apply Core Java concepts.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Get all your Core Java-related questions answered by expert instructors.
Test Series and Assignments
- Topic-Wise Test Series: Assess your understanding with tests after each key Java topic.
- Assignments: Apply your learning through practical assignments focused on Core Java concepts.
Lecture Notes
- Detailed Notes: Access comprehensive notes for every lecture, covering essential Core Java topics.
Projects
- Real-World Projects: Work on live projects to apply Core Java concepts in practical scenarios.
- OOPs and Design Patterns: Implement Object-Oriented Programming principles and design patterns in your projects.
- Best Practices: Learn and apply industry-standard best practices in your Core Java projects.
Certifications
- Dual Certification: Earn two certificates upon course completion and project submission.
- Core Java Completion Certificate: Receive a certificate for successfully completing the Core Java (JSE) course.
- Project Completion Certificate: Get certified for successfully delivering your Core Java project.
Core Java (JSE) Lecture - 1 | Preview |
Core Java (JSE) Lecture - 2 | Preview |
Core Java (JSE) Lecture - 3 | Preview |
Core Java (JSE) Lecture - 4 | Preview |
Core Java (JSE) Lecture - 5 | Preview |
Laptop / Computer Spefiications
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
Java Development Kit (JDK)
Integrated Development Environment (IDE)
Build Tools
Version Control
Database
Documentation
Ready to kick-start your career?
Join Us Now Join Us Now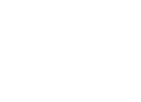
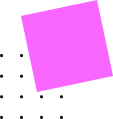