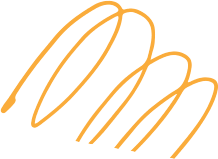
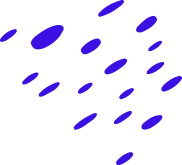
Short Overview
C++ is a cross-platform language that can be used to create high-performance applications. C++ was developed by Bjarne Stroustrup, as an extension to the C language. C++ gives programmers a high level of control over system resources and memory. The language was updated 4 major times in 2011, 2014, 2017, and 2020 to C++11, C++14, C++17, C++20.
- Topics important for C++
- History of C++
- Why C++ is called as C++?
- Why C++?
- Types of C++/Flavors of C++
- Important Differences in flavors of C++
- Compilers and IDEs used for C++
- Syntactical Differences between C & C++
- Important Vocabulary in C++
- What is Cascading?
- Manipulator
- Another Important point in C++
- return type of main () in C and C++
- A simple program in C, Classic C++ and in Modern C++
- Introduction to Object Oriented Programming
- difference between procedure-oriented programming and in Object oriented programming
- Procedure oriented way for designing a project
- Object oriented way for designing a project
- What are objects in object-oriented programming?
- Self-Explanatory Examples for demonstrating the object
- How do we create an object?
- Explanation on Classes and Objects
- Access Specifiers in C++ and Important Points
- General Syntax of declaring a class
- Practical Example for explaining to create classes and it's objects
- Scope Resolution Operator
- Syntax of defining member function
- Syntax of calling a member function
- What will happen in memory when we will create object of the class?
- A programming Challenge in OOPs
- Few Assignments Questions
- Relationship Between C++ and OOPs
- Terminologies used in C++ and OOPs
- Types of Member Functions
- Classification of Programming Languages According to OOP
- Three Pillars of OOPs
- Polymorphism
- Advantage of Polymorphism
- Inheritance
- Encapsulation
- Creating Parametrized Member Functions
- How many ways are there in C++ to initialize data members of the class
- Few Assignments
- Constructor
- The Default Constructor
- Creating Parametrized Constructors
- A practical explanation with a programming challenge
- Function Overloading
- Special Points related to function overloading
- Why we can't overload functions, just on the basis of their return types?
- What is the benefit of overloading?
- Does overloading really exist?
- Constructor Overloading
- Assignment Question
- Copy Constructor
- Reference Variables
- Syntax of Reference variable
- How can we see the actual address of a reference variable?
- Do reference variable really share the address of the variable they refer?
- Pointer v/s Reference Variable
- Argument passing in C++
- Pass by Value
- Pass by address
- Pass by reference using reference variable
- practical examples
- Assignment questions
- Copy Constructor
- Practical Examples of Copy Constructor
- Some Exercises on Copy Constructor
- How many Types of Constructors are there?
- Default Function Arguments
- What is Default Function Arguments?
- Restriction on Default Function Arguments
- Examples of Default Function Arguments
- Restriction on Default Function Argument Call
- ✓ Examples of Restriction on Default Function Argument Call
- Avoiding Constructor Overloading with the help of Default Parametrized constructor
- Destructor
- What is a Destructor?
- When is the destructor called?
- Can we say that a destructor destroys object?
- A program to demonstrate working of a destructor
- Constructor v/s destructor
- A practical example which shows the importance of destructor
- Introduction to member function getline ()
- Syntax of getline ()
- How cout deals with pointer
-
Introduction to Modern C++
-
What is Modern C++
-
Little history of C++ 11/14/17
-
Which Compiler Supports C++17
-
Modern C++ Features
-
Important differences between standard C++ and C++11/14/17
-
What is a Namespace?
-
Important points to remember
-
Namespace std
-
practical example of using namespace
-
New Data types in modern C++
-
The Auto Keyword
-
New Ways of initialization
-
in class initialization
-
range based for loop
-
practical implementation of all new features
- Introduction to Modern C++
- What is Modern C++
- Little history of C++ 11/14/17
- Which Compiler Supports C++17
- Modern C++ Features
- Important differences between standard C++ and C++11/14/17
- What is a Namespace?
- Important points to remember
- Namespace std
- practical example of using namespace
- New Data types in modern C++
- The Auto Keyword
- New Ways of initialization
- in class initialization
- range based for loop
- practical implementation of all new features
- using the keyword static
- What is static in C language?
- few assignments regarding static in C language
- Using static in C++
- What are "static" data members?
- non-static v/s static
- Assignments Questions
- Syntax of call (non-static member function)
- Syntax of call (static member function)
- static member functions
- Assignment questions
- using the keyword "inline" or using "inline" functions
- What are inline functions
- Important points to remember regarding inline functions
- Types of "inline" functions
- The "this" Pointer
- what is "this"?
- What is the data type and size of "this"?
- Accessing object members using "this"
- The for loopIntroduction to Pycharm
- Benefits of using "this"
- benefits of "this"
- practical example of using this
- using the keyword "const"
- What is const
- Points to remember with "const"
- Places where "const" can be used
- pointer to "const"
- Do we have any other way of declaring pointer to const ?
- "const" pointer
- Points to remember
- using "const" pointer to "const"
- uses of "const" keyword
- "const" function argument
- "const" data member
- using the keyword "const"
- Points to remember about "const" data members
- What is Initializer List?
- "const" Member Function
- "const" Objects
- Passing Object as argument to member functions
- why do we have to pass object as argument to member function ?
- In how many ways can we pass an object as argument?
- Passing Object by value
- Passing object by address
- Passing object by reference
- Friend Functions
- A program to demonstrate how to use a friend function?
- Assignment question
- A function being friend of 2 classes
- Friend Classes
- Adding 2 objects of a Class
- Adding 2 objects using friend functions
- Operator Overloading
- What is Operator Overloading?
- Techniques of Overloading Operators
- Syntax of Overloading Operators
- A program to Overload Unary Operator ++ (pre increment) as Member function
- Overloading Post Increment Operator
- Important Points about the Post Increment Operator
- Overloading Operator ++ (Pre-Increment) as friend function
- few assignments question
- Overloading of Binary Operators
- Overloading Operator + as member function
- Overloading Operator + as Friend Function
- Overloading Relational Operators
- Assignment questions
- Rules regarding operator overloading
- Dynamic Memory Allocation
- Dynamic Memory Allocation in C
- Dynamic Memory Allocation in C++
- Syntax of "new"
- Syntax of "delete"
- Assignment question
- Creating Dynamic Objects
- Array of Dynamic Objects
- Dynamic Constructor and Dynamic Destructor
- Inheritance
- What is the benefit of inheriting a class?
- Types of Inheritance
- Syntax of inheriting a class
- Example of single inheritance in "public" mode
- What is "protected"?
- Binding
- Types of Binding
- What is Function Overriding?
- Accessibility rules of base class members in "public" mode of inheritance
- "protected" mode of inheritance
- Accessibility rules of base class members in "protected" mode of inheritance
- "private" mode of inheritance
- Accessibility rules of base class members in "private" mode of inheritance
- Multilevel Inheritance
- Multiple Inheritance
- What is Ambiguity Error?
- Solving Ambiguity Error
- Behavior of Constructor and Destructor in Inheritance
- Calling of Base class Parametrized Constructor in Inheritance
- Constructor calling in Multilevel Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
- What is virtual in C++?
- Polymorphism (Run Time Polymorphism)
- Thumb rule of inheritance
- Late Binding using "virtual"
- Virtual Functions and Multilevel Inheritance
- How Compiler Internally Handles Virtual Functions
- What is the size of an object in C++?
- A practical use of Virtual Functions
- Pure Virtual Functions
- Why Compiler creates a default constructor?
- Why can't we declare constructor as virtual?
- What will happen if we declare our own constructor in a class with Virtual Function?
- Why early binding is fast while late binding is slow?
- Why a pointer of base class cannot access new functions added by the derived class, even though it is pointing to the derived class object?
C++ Programming Lecture - 1.1 | Preview |
C++ Programming Lecture - 1.2 | Preview |
C++ Programming Lecture - 1.3 | Preview |
C++ Programming Lecture - 2.1 | Preview |
C++ Programming Lecture - 2.2 | Preview |
C++ Programming Lecture - 3.1 | Preview |
C++ Programming Lecture - 3.2 | Preview |
C++ Programming Lecture - 3.3 | Preview |
From Fundamentals to Advanced C++ Programming
- C++ Basics to Advanced: Master C++ programming from fundamental concepts to advanced topics.
- Zero to Hero: Transition from a beginner to an expert C++ developer.
- Comprehensive Coverage: Learn everything from object-oriented programming (OOP) to templates, STL, and advanced memory management.
Live Lectures
- 80+ Hours: Engage in live, interactive sessions led by seasoned C++ developers.
- Hands-on Practice: Reinforce your C++ skills through practical coding exercises during live sessions.
- Practice Sessions: Participate in focused practice sessions to fully grasp and apply C++ concepts.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Resolve all your C++ programming queries with help from expert instructors.
Test Series and Assignments
- Topic-Wise Test Series: Evaluate your understanding with tests after each significant topic.
- Assignments: Apply your knowledge through practical coding assignments focused on C++.
Lecture Notes
- Detailed Notes: Access comprehensive notes for every lecture, covering crucial C++ programming topics.
Projects
- Real-World Projects: Implement C++ concepts in live projects to solve real-world problems.
- OOPs and Design Patterns: Apply Object-Oriented Programming principles and design patterns in your C++ projects.
- Best Practices: Learn and adopt industry-standard best practices in your C++ programming projects.
Certifications
- Dual Certification: Receive two certificates upon successful course completion and project submission.
- C++ Completion Certificate: Obtain a certificate for completing the C++ programming course.
- Project Completion Certificate: Get certified for successfully completing your C++ project.
Computer / Laptop Requirments
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
C++ Compiler
Integrated Development Environment (IDE)
Version Control
Documentation
Libraries
- Download Boost
- STL (Standard Template Library): Comes with most C++ compilers, including GCC, Clang, and MSVC.
Ready to kick-start your career?
Join Us Now Join Us Now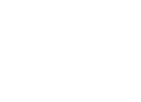
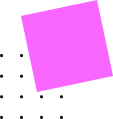