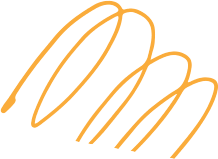
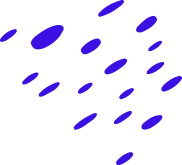
Short Overview
Data structures are essential for organizing, managing, and storing data efficiently. In C++, data structures enable you to perform operations such as searching, sorting, and manipulating data effectively.
- Course Overview
- Introduction of Data Structure & Algorithm
- Types of Data Structure
- What is Stack?
- Stack Implementations
- Application of Stack
- Evaluation of Prefix and Postfix Expression
- Conversion of Infix to Postfix
- Conversion of Infix to Prefix
- What is Queue?
- Queue Implementations
- Linear Queue V/s Circular Queue
- Application of Queue
- What is Linked List?
- Types of Linked List
- Implementation of Singly Linked List
- Implementation of Circular Linked List
- Implementation of Doubly linked list
- Implementation of Dynamic Stack
- Application of Linked List
- Addition of Polynomial using Linked List
- What is Tree?
- Binary Tree
- Complete Binary Tree
- Extended Binary Trees
- Array and Linked Representation of Binary trees
- Traversing Binary trees
- Representation of Binary Tree
- Threaded Binary trees
- Binary Search Tree
- Implementation of BST
- Preorder Traversal
- Inorder Traversal
- Postorder Traversal
- Tree Traversal Algorithms
- Heap
- AVL Tree
- Threaded Binary Tree
- What is Graph?
- Representation of Graphs
- What is Graph Traversal ALgorithm
- What is Shortest path
- Dijkstra's Algorithm
- Warshall's Algorithm
- What is spanning tree
- Minimal Spanning Tree
- Prim's Algorithm
- Kruskal Algorithm
- Recursion
- Format of Recursive Function
- Application of Recursion
- Asymptotic Notation
- Big O Notation
- Omega-Ω notation
- Theta-𐐃 notations
- Guidelines for Asymptotic Analysis
- Sorting
- Bubble Sort
- Selection Sort
- Insertion Sort
- Quick Sort
- Merge Sort
- Heap Sort
- Linear Search
- Sequential Search
- Binary Search
- What is Hashing?
- What is Collision?
- Collision Resolution Technique
- What is Linear Probing?
- What is Bucket Technique?
- What is Chaining Technique
- What is STL
- Importance of STL
- Collision Resolution Technique
-
Three Member's of STL -
- Containers
- Iterators
- Algorithms
From Fundamentals to Advanced Data Structures in C++
- Basics to Advanced: Master data structures using C+ programming, starting from basic concepts to advanced techniques.
- Zero to Hero: Progress from a beginner to an expert in implementing data structures using C++.
- Comprehensive Coverage: Learn everything from arrays, linked lists, stacks, and queues to trees, graphs, and hash tables.
Online/Offline Lectures
- 50+ Hours: Attend live, interactive sessions taught by experienced instructors in data structures and C++ programming.
- Hands-on Practice: Enhance your understanding of data structures by coding during live sessions.
- Practice Sessions: Participate in dedicated sessions to solidify your grasp on complex data structures.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Get all your questions answered with support from expert instructors.
Test Series and Assignments
- Topic-Wise Test Series: Evaluate your understanding with tests after each major data structure.
- Assignments: Apply your learning through practical coding assignments focused on data structures using C++.
Lecture Notes
- Detailed Notes: Access comprehensive notes for every lecture, covering critical data structure concepts.
Certifications
- Data Structures in C++ Completion Certificate: Earn a certificate for mastering data structures using C++.
Computer / Laptop Requirments
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
C++ Compiler
- GCC (GNU Compiler Collection): A widely used open-source compiler for C++.
- Clang: A compiler for C++ that is part of the LLVM project.
- Microsoft Visual Studio: Includes the MSVC (Microsoft Visual C++) compiler for Windows.
Integrated Development Environment (IDE)
- Code::Blocks: A free C/C++ IDE with support for various compilers.
- Dev-C++: An open-source IDE for C/C++ programming.
- Visual Studio Code: A versatile code editor that supports C++ development with extensions.
Text Editors
- Sublime Text: A lightweight text editor with support for C++ syntax highlighting through plugins.
- Notepad++: A text editor with C++ syntax highlighting and support for code editing.
Version Control System
Documentation and Resources
- The C++ Programming Language by Bjarne Stroustrup: A comprehensive book by the creator of C++.
Online Compilers (for quick testing)
Ready to kick-start your career?
Join Us Now Join Us Now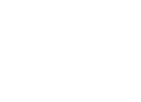
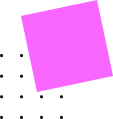