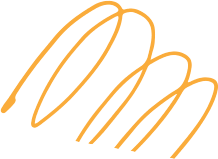
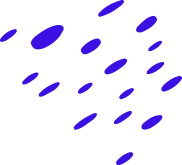
Short Overview
Data Structure in java is defined as the collection of data pieces that offers an effective means of storing and organising data in a computer. Linked List, Stack, Queue, and arrays are a few examples of java data structures.
- Course Overview
- Introduction of Data Structure & Algorithm
- Types of Data Structure
- Introduction of Stack
- Terminologies related to Stack
- Diagrammatic representation of Stack
- Implementing Stack in Java Language
- First Version of Stack ( Array )
- Modified version of Stack using Loop
- Implementing Stack Class in Java Language
- Pseudocode for the methods Push( ) & Pop( )
- What is Expression ?
- Type of Expression ?
- Precedence of Operator
- Evaluating Postfix and Prefix Expression
- Pseudocode for evaluating a Postfix Expression
- Implementing a Class for evaluating a Postfix Expression
- Remaining methods of evaluation of Postfix Expression
- Pseudocode for evaluating a Prefix Expression
- Implementing a Class for evaluating a Prefix Expression
- Remaining methods of evaluation of Prefix Expression
- Converting Infix to Postfix
- Pseudocode for converting Infix to Postfix
- Implementation of converting Infix to Postfix using Stack
- Converting Infix to Prefix
- Pseudocode for converting Infix to Prefix
- Implementation of converting Infix to Prefix using Stack
- Converting Parenthesised Infix to PostFix
- Pseudocode for converting Parenthesised Infix to PostFix
- Introduction of Queue
- Diagrammatic view of Queue
- What is Queue and it's real world examples
- Pseudocode for the methods insert( ) & remove( )
- Implementation of Linear Queue in Java
- Drawback of Linear Queue
- Solution for the drawback of Linear Queue
- Introduction of Circular Queue
- Theory of Circular Queue
- Pseudocode for the insert() & remove()
- Implementation of Circular Queue in Java
- Drawbacks of arrays
- How LinkedList over come drawbacks of array
- What is LinkedList
- Advantages of LInkedList
- Disadvantages of LinkedList
- Implementing of LinkedList
- Implementing a LinkedList using Class in Java
- Displaying nodes of a Linked List
- Deleting First node from a LinkedList
- Program to make method for search_Node()
- Deleting last node from a Linked List
- Deleting any node from Linked List
- Program to make method called insert()
- Introduction of Circular Linked List
- Implementing a Circular Linked List
- Deletion in Circular Linked List
- Deleting first node of Circular Linked List
- Deleting Last node of Circular Linked List
- Program to make function del_Any( ) for Circular Linked List
- Introduction of Doubly Linked List
- Implementation a Doubly Linked List
- Deleting first node of Doubly Linked List
- Deleting Last node of Circular Linked List
- Explanation of Application of Linked List
- Diagrammatic view of Dynamic Stack
- Implementation of Dynamic Stack
- Program to make function delAny( ) fro Doubly Linked List
- Implementing Queue using Linked List
- Addition of Polynomial using Linked List
- Implementation for adding 2 Polynomial
- Introduction of Non-Linear Data Structure
- Examples and types of Non-Linear Data Structure
- Introduction of Tree Data Structure and Binary Tree
- Terminologies used in tree with examples
- Introduction of Binary Search Tree and it’s examples
- Representation of Binary Search Tree using array and linked List
- Important points of Array and Linked List representation of Binary Tree
- Advantages of Array and Linked List representation of BST
- Disadvantages of Array and LinkedList repress of BST
- Implementation of BST
- Explanation of Deletion in Binary Search Tree
- Explanation of method search ( ) in Binary Search Tree
- Implementation of Deletion in Binary Search Tree
- Introduction of Tree Traversal Algorithms
- Explanation of Preorder Traversal with PseudoCode
- Implementation of Preorder Traversa
- Explanation of Inorder Traversal
- PseudoCode for Inorder Traversal
- Implementation of Inorder Traversal
- Explanation of Postorder Traversal
- PseudoCode for Postorder Traversal
- Implementation of Postorder Traversal
- Identification of PreOrder , InOrder and PostOrder traversal
- Introduction of Heap
- Creating a Max Heap
- Code for Creating a Heap in Java
- Insertion in Heap
- Deleting Root in the Heap
- Pseudocode for deleting root of the Heap
- Constructing a binary root of the Heap
- Constructing a binary tree using Traversal Order
- What is AVL Tree
- What is the benefit of a BST over an Array
- What is Balanced Factor
- How do we create an AVL Tree
- Draw an AVL Tree
- Types of Rotation
- What is double rotation
- Draw an AVL Tree with single rotation and double rotation
- Two special Rules of rotation
- Implementing AVL Tree and its Functions
- Method nodeHeight( ) & balanceFactor( )
- Method LLRotation( ) , RRRotation( ) , LRRotation( ) & RLRotation( )
- Introduction of Threaded Binary Tree
- Types of Binary Tree
- One way InOrder TBT
- Two way InOrder TBT
- Two way InOrder TBT with a header node
- PseudoCode for one way TBT
- Implementation of One way TBT
- What is B-Tree ?
- What is Multiway Tree ?
- Properties of B-Tree
- Constructing a B-Tree
- How do we Handle overFlow in B-Tree ?
- Introduction of Graph
- Difference between Graph and Tree
- Terminologies used in Graph
- Ways of representing a graph in memory
- What is Adjacency Matrix
- What is Path Matrix
- Ways of deriving Path Matrix
- Deriving Path matrix using Adjacency matrix
- Code for Adjacency Matrix
- Calculating Path matrix using warshall's Algorithm
- Implementation of Warshall's Algorithm
- Basic concepts of 1d and 2d array
- Introduction of Linked representation of a graph
- Implementation of Method addVertex( ) , insertEdge( ) , findVertex( ) & display ( )
- Program : Write method for deleting Vertex & deleting Edge
- What is Graph Traversal Algorithms
- Explanation of BFS
- Explanation of DFS
- PseudoCode of DFS
- Implementation of DFS Traversal Algorithm
- What is Shortest Path
- Warshall's Algorithm for calculating shortest path matrix
- Implementation of Warshall's Shortest path matrix
- Dijikstra's Algorithm for calculating shortest path between 2 vertices
- What is spanning Tree ?
- What is Minimum cost spanning Tree ?
- What is Prism's Algorithms
- Explanation of Prism's Algorithm
- Implementation of Prism Algorithm
- Kruskal's Algorithm for calculating minimum cost spanning tree
- What is Recursion ?
- How Recursion is used in programming ?
- Prerequisites of Recursive methods
- Example Question of Recursion
- How memory and method calls are managed on run time ?
- Tracing Recursion Functions
- Explanation of Types of Recursion
- Recursion v/s Iteration
- Some popular examples of Recursion
- Factorial using Recursion
- Displaying Linked List
- Complexity of Algorithm
- How do we calculate the time taken by the Algorithm
- Examples of Time Complexity
- Linear Search and it's Implementation
- Complexity analysis of Linear Search
- Binary Search and it's Implementation
- Complexity analysis of Binary Search
- Bubble Sort Algorithm
- Implementation of Bubble Sort
- Complexity of Bubble sort
- Selection sort and it's Implementation
- Complexity of Selection Sort
- Insertion sort and it's Implementation
- Complexity Analysis of Insertion Sort
- Comparison Between Bubble sort , Insertion sort and selection sort
- Merge sort algorithm ( Divide and Conquer method )
- Implementation of Merge Sort
- Complexity analysis of merge sort
- What is Quick Sort Algorithm
- Implementation of Quick Sort Algorithm
- Complexity analysis of Quick Sort
- What is Heap Sort ?
- Implementation of Heap Sort
- Complexity analysis of Heap Sort
- What is Priority Queue ?
- Linked List Implementation of Priority Queue
- Array Implementation of Priority Queue
- Best way of array implementation of Priority Queue
- What is Hashing ?
- What is Collision ? And collision resolution techniques
- What is Linear probing , it's methods implementation and it's drawback
- What is Bucket Technique ? & chaining technique ?
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
Ready to kick-start your career?
Join Us Now Join Us Now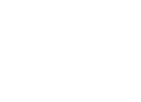
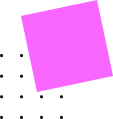