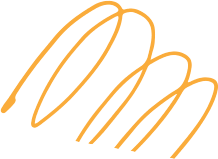
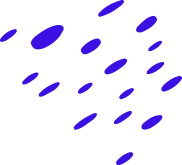
Short Overview
Hibernate is an Object-Relational Mapping (ORM) solution for JAVA. It is an open source persistent framework created by Gavin King in 2001. It is a powerful, high performance Object-Relational Persistence and Query service for any Java Application
- Course Overview
- What Is Hibernate ?
- What Is JDBC And What Are Problems With JDBC ?
- What Is ORM ?
- Advantages Of Hibernate
- Why Should We Learn Hibernate ?
- Architecture Of Hibernate
- Layers In Hibernate
- Files Needed For An Hibernate Application
- Details Of These Files
- Three Important Objects Of HIBERNATE
- Hibernate’s Built-In Objects
- Three Special Objects Of Hibernate
- Details Of These Three Objects
- Creating These Three Objects
- Softwares Required
- Downloading Hibernate Jar Files
- What is get () ?
- How get() works ?
- What is load() ?
- How load() works ?
- Why two methods ?
- The difference between get() and load() ?
- Different ways of saving object
- How save() works ?
- What is transaction?
- How hibernate handles transaction?
- Transaction methods
- Using The persist() Method
- Save() V/s persist()
- Using The update() Method
- Two Ways To Update A Record
- Points To Remember
- Using The saveOrUpdate() Method
- Saving Persistent Object To Database
- Using The merge() Method
- Different Scenarios
- Differences with update()
- Deleting The Object
- Points To Remember
- States Of an Object
- Difference Between These States
- Different Ways To Change State
- Points To Remember
- Problems With Our Current Approach
- Introduction To Layered Architecture
- The Three Layers Involved
- Introduction To DAO Pattern
- Example
- What Is hbm2ddl.auto ?
- Original values
- New values added
- Example
- What Is Generator Tag ?
- List Of All Predefined Generators
- The Assigned Generator
- The Increment Generator
- The Sequence Generator
- The Identity Generator
- Identity V/s Increment
- The Hilo Generator
- The Algorithm Used By Hilo
- A Special Point
- The Sequence-Hilo Generator
- Example
- Benefits And Drawbacks
- The Native Generator
- What Is HQL ?
- Why HQL ?
- HQL V/s SQL
- HQL Syntax
- Executing SELCECT Queries
- Selecting Full Object
- Selecting Partial Object
- Selecting Only One Column
- Using As and Where
- Introduction To EL
- What Is Parameter Binding ?
- Different Ways Of Parameter Binding
- Using Named Parameters
- The setParameter() Method
- The setString() Method
- The setProperties() Method
- Using Positional Parameters
- Using ORDER BY
- Using GROUP BY
- Executing UPDATE
- Executing DELETE
- Executing INSERT
- What Is Criteria API ?
- Criteria Limitations
- Important Classes Of Criteria
- Criteria Methods
- What Is JPA Criteria API ?
- Important Interfaces JPA Criteria API
- Steps Required To Use JPA Criteria API
- Selecting Full Object
- Selecting One Column
- Selecting Partial Object
- Applying Aggregate Functions
- Selecting Number Of Records
- Selecting Max
- Selecting Avg
- Selecting Sum
- Applying Order By
- Applying Group By
- Filtering Using Having
- Restricting Rows Using Where
- New Feature In JPA 2.1
- Updating Records
- Deleting Records
- What Is Native SQL ?
- Different Ways To Execute Native SQL
- Selecting Full Object
- Selecting Partial Object
- Parameter Binding
- Inserting Records
- Using Positional Parameters
- Using Named Parameters
- New Way Of Writing Native SQL
- Selecting Full Object
- Selecting Partial Object
- Parameter Binding
- Inserting Records
- Using Positional Parameters
- Using Named Parameters
- What Is Named Query ?
- Named Query Advantages
- Named Query For HQL
- Named Query For SQL
- What Is Pagination?
- How Hibernate Supports Pagination ?
- Fetching First Page
- Fetching Multiple Pages
- Introduction To RelationShips
- Types Of RelationShips
- What Is One To Many ?
- Achieving One To Many
- Inserting Records In One To Many
- Selecting Records From Join Tables
- Selecting Single Record
- Selecting All Records
- Fetching Strategies
- Deleting Records From Join Tables
- Deleting Only Child Record
- Deleting Parent And Child Records
- Updating Records From Join Tables
- Updating Only Child Record
- Updating Only Parent
- Updating Both Parent And Chils
- What Is Many To One
- Achieving Many To One
- Inserting Records In Many To One
- Selecting Records From Join Tables
- Selecting Single Record
- When Hibernate Fetches Parent Record ?
- Selecting All Records
- Deleting Records From Join Tables
- Deleting Only Child Record
- What About Deleting Parent ?
- Updating Records From Join Tables
- Updating Only Child Record
- Updating Only Parent
- Updating Both Parent And Chils
- Bi-Directional RelationShips
- Requirements
- Insertion In Bidirectional
- Selecting Records In Bidirectional
- Updating Records In Bidirectional
- Deleting Records In Bidirrectional
- Many To Many Relationship
- Requirements
- Insertion From Course To Srudent
- Insertion From Student To Course
- Selecting Records From Many To Many
- Selecting Single Record
- Selecting All Records
- Deleting Records From Many To Many
- Deleting From Only JOIN Table
- Deleting From Other Table
- Want Is One To One ?
- Important Tags
- New Concept Called Shared Primary Key
- Insertion In One To One
- Selecting Records
- Introduction
- What Is Caching ?
- Types Of Cache
- First Level Cache
- Removing Objects From Cache
- Second Level Cache
- The Process Internally
- Popular Implementations
- Using EHCache
- What Are Annotations ?
- Benefits Of Annotations
- Most Common Annotations
- Composite Primary Key
Hibernate Development Lecture - 1.1 | Preview |
Hibernate Development Lecture - 1.2 | Preview |
Hibernate Development Lecture - 1.3 | Preview |
Hibernate Development Lecture - 2.1 | Preview |
Hibernate Development Lecture - 2.2 | Preview |
Hibernate Development Lecture - 3.1 | Preview |
Hibernate Development Lecture - 3.2 | Preview |
Hibernate Development Lecture - 3.3 | Preview |
Hibernate Development Lecture - 4.1 | Preview |
Hibernate Development Lecture - 4.2 | Preview |
Hibernate Development Lecture - 4.3 | Preview |
Hibernate Development Lecture - 5.1 | Preview |
Hibernate Development Lecture - 5.2 | Preview |
Hibernate Development Lecture - 5.3 | Preview |
From Basics to Advanced Hibernate
- Basics to Advanced: Master Hibernate ORM from basic concepts to advanced techniques in persistence and data handling.
- Zero to Hero: Transform from a beginner to an expert in using Hibernate for database management.
- Comprehensive Coverage: Learn everything from setting up Hibernate, mapping entities, and handling relationships to advanced queries and optimization techniques.
Live Lectures
- 60+ Hours: Participate in live, interactive sessions with experienced Hibernate professionals.
- Hands-on Practice: Apply Hibernate concepts during live coding sessions and practice implementations.
- Practice Sessions: Engage in dedicated sessions to master the use of Hibernate in real-world scenarios.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Get all your questions answered by expert instructors.
Test Series and Assignments
- Topic-Wise Test Series: Evaluate your understanding with tests after each major Hibernate topic.
- Assignments: Apply your learning through practical coding assignments focused on Hibernate frameworks.
Lecture Notes
- Detailed Notes: Access comprehensive notes for every lecture, covering key Hibernate concepts and best practices.
Certifications
- Hibernate Completion Certificate: Earn a certificate for mastering Hibernate ORM.
Computer / Laptop Requirments
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
Java Development Kit (JDK)
- JDK 8 or later: Required for running Hibernate applications. Ensure compatibility with your Hibernate version.
Integrated Development Environment (IDE)
- Eclipse: A popular IDE with plugins for Hibernate development.
- IntelliJ IDEA: A powerful IDE with built-in support for Hibernate.
- NetBeans: An IDE with support for Java EE and Hibernate.
Hibernate Framework
- Hibernate ORM: The core ORM framework for Java. Add Hibernate to your project via Maven or Gradle:
- Maven:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.6.12.Final</version>
</dependency> - Gradle:
implementation 'org.hibernate:hibernate-core:5.6.12.Final'
- Maven:
Database
- MySQL: A commonly used relational database with Hibernate.
- PostgreSQL: Another popular choice for Hibernate applications.
- Oracle Database: For applications that use Oracle as their RDBMS.
Database Drivers
- MySQL Connector/J: JDBC driver for MySQL.
- PostgreSQL JDBC Driver: JDBC driver for PostgreSQL.
- Oracle JDBC Driver: JDBC driver for Oracle Database.
Build Tools
- Maven: For managing project dependencies and building Java applications.
- Gradle: An alternative to Maven, used for dependency management and build automation.
Version Control System
Database Management Tools
- DBeaver: A universal database tool that supports various RDBMS, including MySQL, PostgreSQL, and Oracle.
- HeidiSQL: A tool for managing MySQL and PostgreSQL databases.
Documentation and Resources
- Hibernate Documentation: Official guide for Hibernate ORM.
- Hibernate Forums: Community forums for asking questions and finding answers.
Configuration and Utility Tools
- HikariCP: A high-performance JDBC connection pool.
- Apache Commons DBCP: Another option for database connection pooling.
Ready to kick-start your career?
Join Us Now Join Us Now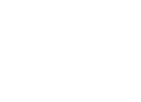
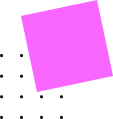