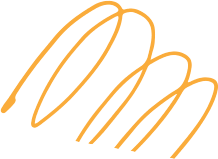
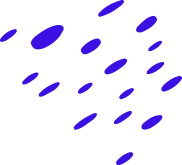
Short Overview
Node.js is an open-source and cross-platform JavaScript runtime environment. It is a popular tool for almost any kind of project. Node.js runs the V8 JavaScript engine, the core of Google Chrome, outside of the browser. This allows Node.js to be very performant.
- Introduction
- Who this course is for
- How Node.JS Works
- Node V8 Engine
- Installation Node.JS
- What is REPL - Read Evaluate Print Line
- Loading Files - Using REPL
- Process Objec
- Global Namespace
- Buffers
- Understanding Callbacks
- Introduction
- Making use of NPM - Node Package Manager
- Publishing node modules
- Module Caching
- Module Cashing - Overcoming the limitations
- Module Patterns
- Events and EventEmitter
- EventEmitter and Inheritance
- Creating your own EventEmitter
- Creating Classes in ES6
- ES6 Classes and EventEmitter
- Introduction to Streams
- Readable Streams
- Writeable Streams
- Piping
- Chaining
- Set Encoding
- Additional Methods of Readable Streams
- Duplex Streams
- Introduction
- Promises
- Generators
- Resolving Callback Hell
- How a Web Server works
- Creating our WebServer
- Sending HTML content as response from WebServer
- Serving Static Contents
- Post data to Node Web Server
- Handling Querystring
- Introduction to Express
- Installing Express
- Creating a Web Server using Express
- Adding routes to Express App
- Working with Express Templates
- Working with partials
- Sharing content across routes
- Organizing our routes
- Making use of Express Generator
- Express Middleware
- Developing a project using Express
- Apply All the Concepts Learnt till Now
- Understanding assets of our Express Web Application
- Structuring our express web application
- Introduction to Database
- Download and installing MySQL and Workbench on windows
- Connecting MySQL with our Express Application
- Introduction to NoSQL Database
- Installing to MongoDB Locally
- Working with MongoDB and Mongoose
- Using Mongolab - MongoDB as a Service
- Setting up our Authentication Application
- Creating User Model in Mongoose
- Developing Strategies - I - Login Strategy
- Developing Strategies - II - Signup Strategy
- Creating Routes
- Creating our Login/Signup Layout and Finishing the Application
From Basics to Advanced Node.js
- Basics to Advanced: Learn Node.js development from fundamental concepts to advanced techniques.
- Zero to Hero: Evolve from a beginner to an expert in building scalable server-side applications with Node.js.
- Comprehensive Coverage: Understand everything from Node.js basics, asynchronous programming, and APIs to advanced topics like microservices and performance optimization.
Live Lectures
- 45 Hours: Attend live, interactive sessions with experienced Node.js developers.
- Hands-on Practice: Apply Node.js concepts during live coding sessions and work on real-world projects.
- Practice Sessions: Engage in dedicated practice sessions to refine your skills in Node.js development.
Doubt Solving
- Unlimited Doubt Clearing Sessions: Get all your questions answered with support from expert instructors.
Test Series and Assignments
- Topic-Wise Test Series: Evaluate your understanding with tests after each major Node.js topic.
- Assignments: Apply your learning through practical coding assignments and projects using Node.js.
Lecture Notes
- Detailed Notes: Access comprehensive notes for every lecture, covering key Node.js concepts and best practices.
Projects
- Real-World Projects: Work on live projects to implement and deploy Node.js applications in real-world scenarios.
- API Development: Learn to create and manage RESTful APIs using Node.js and Express.js.
- Performance Optimization: Understand how to optimize Node.js applications for better performance and scalability.
Certifications
- Dual Certification: Receive two certificates upon successful completion of the course and projects.
- Node.js Completion Certificate: Earn a certificate for mastering Node.js development.
- Project Completion Certificate: Get certified for successfully completing your Node.js projects.
Computer / Laptop Requirments
- System With Minimum I3 Processor Or Better
- At Least 4 Gb Of Ram
Text Editor/IDE
- Visual Studio Code: A widely used code editor for JavaScript development.
- WebStorm: A powerful IDE designed for JavaScript development.
- Sublime Text: A lightweight text editor for quick coding sessions.
Node.js and npm
- Node.js: JavaScript runtime environment used for building the server-side in Express.
- npm (Node Package Manager): Comes installed with Node.js. Used to manage packages like Express.
- Yarn (optional): An alternative package manager to npm.
Express.js
- Express.js: A minimal and flexible Node.js web application framework. Installed via npm:
npm install express --save
MongoDB
- MongoDB: A NoSQL database used for storing data in JSON-like documents.
- MongoDB Atlas (Optional): A cloud-hosted version of MongoDB, ideal for production environments.
- Mongoose: A MongoDB object modeling tool designed to work in an asynchronous environment. Installed via npm:
npm install mongoose
Version Control System
Browser
- Google Chrome: A modern browser with excellent developer tools for debugging Node.js APIs.
- Mozilla Firefox: Another browser with helpful developer tools.
Postman or Insomnia (API Testing Tools)
- Postman: A popular tool for testing RESTful APIs.
- Insomnia: Another powerful API testing tool to interact with Node.js backends.
Database Client
- MongoDB Compass: A graphical user interface (GUI) to interact with your local or remote MongoDB databases.
- Robo 3T: Another popular GUI tool for MongoDB, providing a rich interface for database management.
REST Client Library (Optional)
- Axios: A promise-based HTTP client for the browser and Node.js. Can be installed via npm:
npm install axios
Nodemon (Optional)
- Nodemon: A utility that monitors for any changes in your Node.js application and automatically restarts the server. Install it globally with:
npm install -g nodemon
Security Libraries (Optional)
- Helmet: Helps secure Express apps by setting various HTTP headers. Installed via npm:
npm install helmet
- bcrypt: A library for hashing passwords before storing them in the MongoDB database. Installed via npm:
npm install bcrypt
Environment Variables (Optional)
- dotenv: A module that loads environment variables from a
.env
file intoprocess.env
. Useful for sensitive data like API keys or database URIs. Installed via npm:npm install dotenv
Deployment Tools
- Heroku: A cloud platform for deploying Node.js and Express apps.
- DigitalOcean or AWS EC2: For more flexible server deployment options.
- Docker: For containerizing your Node.js applications and MongoDB instances.
Ready to kick-start your career?
Join Us Now Join Us Now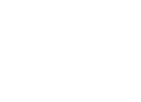
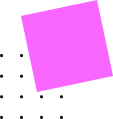